Creating Mailing list Application

In this time I will show, how to make a simple mailing list application with VB. The application allows the entry of information (names and addresses) to build a mailing list. An added feature is a timer that keeps track of the time spent entering addresses. After each entry, rather than write the information to a database (as we would normally do), the input information is simply displayed in a Visual Basic message box. We present this example to illustrate the steps in building an application. If you feel comfortable building this application and understanding the corresponding code, you probably possess the Visual Basic skills needed to proceed with this course.
1. Start a new project. Place two frames on the form (one for entry of address information and one for the timing function). In the first frame, place five labels, five text boxes, and two command buttons. In the second frame, place a label control, a timer control and three command buttons. Remember you need to ‘draw’ controls into frames. Resize and position controls so your form resembles this:
2. Set properties for the form and controls (these are just suggestions – make any changes you might like):
Form1:
Name frmMailingList
BorderStyle 1-Fixed Single
Caption Mailing List Application
Frame1:
Name fraMail
Caption Address Information
Enabled False
Label1:
Caption Name
Label2:
Caption Address
Label3:
Caption City
Label4:
Caption State
Label5:
Caption Zip
Text1:
Name txtInput
Index 0 (a control array)
TabIndex 1
Text [blank it out]
Text2:
Name txtInput
Index 1
TabIndex 2
Text [blank it out]
Text3:
Name txtInput
Index 2
TabIndex 3
Text [blank it out]
Text4:
Name txtInput
Index 3
TabIndex 4
Text [blank it out]
Text5:
Name txtInput
Index 4
TabIndex 5
Text [blank it out]
Command1:
Name cmdAccept
Caption &Accept
TabIndex 6
Command2:
Name cmdClear
Caption &Clear
Frame1:
Name fraTime
Caption Elapsed Time
Label6:
Name lblElapsedTime
Alignment 2-Center
Backcolor White
BorderStyle 1-Fixed Single
Caption 00:00:00
FontBold True
FontSize 14
Timer1:
Name timSeconds
Enabled False
Interval 1000
Command3:
Name cmdStart
Caption &Start
Command4:
Name cmdPause
Caption &Pause
Enabled False
Command5:
Name cmdExit
Caption E&xit
When done, the form application should appear something like this:
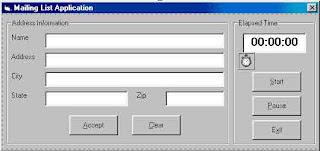
3. Put these lines in the General Declarations area of the code window:
Option Explicit
Dim ElapsedTime As Variant
Dim LastNow As Variant
4. Put these lines in the Form_Load event procedure:
Private Sub Form_Load()
ElapsedTime = 0
End Sub
5. Put this code in the txtInput_KeyPress event procedure:
Private Sub txtInput_KeyPress(Index As Integer, KeyAscii As Integer)
'Check for return key
If KeyAscii = vbKeyReturn Then
If Index = 4 Then
cmdAccept.SetFocus
Else
txtInput(Index + 1).SetFocus
End If
End If
'In Zip text box, make sure only numbers or backspace pressed
If Index = 4 Then
If (KeyAscii >= Asc("0") And KeyAscii <= Asc("9")) Or KeyAscii = vbKeyBack Then
Exit Sub
Else
KeyAscii = 0
End If
End If
End Sub
Note the line beginning with ‘If (KeyAscii >= Asc(“0”) And ...’ is so long that the word processor wraps the line around at the margin. Type this as one long line, not two separate lines or review the use of the Visual Basic line continuation character (_). Be aware this happens quite often in these notes when actual code is being presented..
6. Put this code in the cmdAccept_Click event procedure:
Private Sub cmdAccept_Click()
Dim S As String, I As Integer
'Accept button clicked - form label and output in message box
'Make sure each text box has entry
For I = 0 To 4
If txtInput(I).Text = "" Then
MsgBox "Each box must have an entry!", vbInformation + vbOKOnly, "Error"
Exit Sub
End If
Next I
S = txtInput(0).Text + vbCrLf + txtInput(1).Text + vbCrLf
S = S + txtInput(2).Text + ", " + txtInput(3).Text + " " + txtInput(4).Text
MsgBox S, vbOKOnly, "Mailing Label"
Call cmdClear_Click
End Sub
7. Put this code in the cmdClear_Click event procedure:
Private Sub cmdClear_Click()
Dim I As Integer
'Clear all text boxes
For I = 0 To 4
txtInput(I).Text = ""
Next I
txtInput(0).SetFocus
End Sub
8. Put this code in the cmdStart_Click event procedure:
Private Sub cmdStart_Click()
'Start button clicked
'Disable start and exit buttons
'Enabled pause button
cmdStart.Enabled = False
cmdExit.Enabled = False
cmdPause.Enabled = True
'Establish start time and start timer control
LastNow = Now
timSeconds.Enabled = True
'Enable mailing list frame
fraMail.Enabled = True
txtInput(0).SetFocus
End Sub
9. Put this code in the cmdPause_Click event procedure:
Private Sub cmdPause_Click()
'Pause button clicked
'Disable pause button
'Enabled start and exit buttons
cmdPause.Enabled = False
cmdStart.Enabled = True
cmdExit.Enabled = True
'Stop timer
timSeconds.Enabled = False
'Disable editing frame
fraMail.Enabled = False
End Sub
10. Put this code in the cmdExit_Click event procedure:
Private Sub cmdExit_Click()
'Exit button clicked
End
End Sub
11. Put this code in the timSeconds_Timer event procedure:
Private Sub timSeconds_Timer()
'Increase elapsed time and display
ElapsedTime = ElapsedTime + Now - LastNow
lblElapsedTime.Caption = Format(ElapsedTime, "hh:mm:ss")
LastNow = Now
End Sub
12. Save the application. Run the application. Make sure it functions as designed. Note that you cannot enter mailing list information unless the timer is running.
wah bagusnya kalau bisa dapat ilmu gini